How to Use Bash Associative Arrays to Store Data
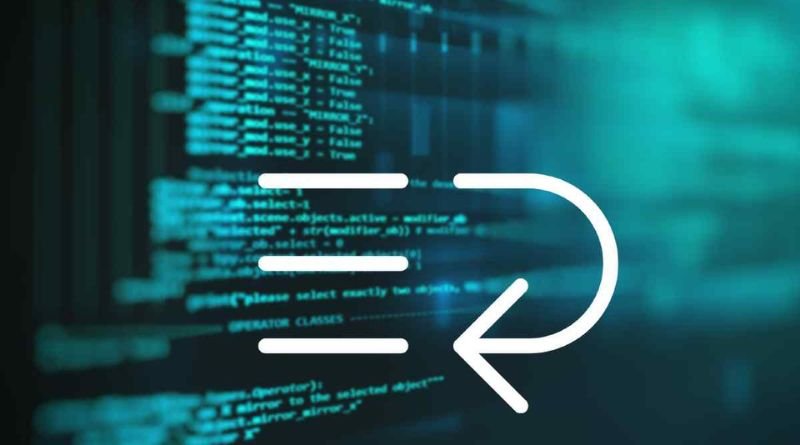
Associative arrays, also known as hashes, are unordered collections of values in which each value has one and only one key to associate it with. In Bash, associative arrays are implemented as normal arrays except that their values may contain complex data structures like strings and even other arrays. Here we’ll discuss how to use Bash associative arrays to store data, how to retrieve data from them, and how to use conditional logic to perform operations on their contents.
Intro to Bash script
In this tutorial, we’ll explore the Bash associative array data type and its use in programming. It’s a handy tool for any programmer because it stores key-value pairs together. Each element is accessed with an index number, called a subscript. Associative arrays can be defined as follows my_array=(firstname Luke lastname Skywalker)
Getting Started with Variables
Variables in Bash can be used to store information and reuse it later in your script. Variables are given a name, like firstname or pi, and each one has an associated value. For example, the firstname variable could be assigned the string ‘John’ while the pi variable would be set to 3.14. Variable values can also be replaced with strings, numbers or operators if desired.
Working with Strings in Bash
Strings are an important part of any programming language. They can be used for storing things like text or characters, and they’re the basic building block for larger structures like lists or sets. However, there are two drawbacks to using strings: Strings can’t be modified after initialization, and there’s no support for adding or removing items from a string once it’s created. In this post, we’ll take a look at associative arrays in Bash. An associative array is similar to a list in that you can use it to store multiple values. The key difference is that instead of ordering those values by position, they’re ordered by their associated value (hence the name). Associative arrays also have some other useful features that make them even more powerful than lists
Using Array Syntax in a Script
If you want to use associative arrays in a script, here is some syntax. You would just substitute $1 with the variable that you want assigned a value, $2 with the variable that you want assigned another value, and so on.
## Function: Takes two arguments, $user and $gift, and prints out an appropriate greeting. ## Greeting() {
printf Hi %s! Welcome back to our store! What did you bring us today? ${1} ${2}
Iterating Through an Array
When working with data in Bash, you can use associative arrays. An associative array is an unordered list of variables, where each variable has a name and a value associated with it. The syntax for creating one is ARRAY =(variable1 variable2);. Each variable needs to be assigned a string or number when the array is created.
Creating an Associative Array
Arrays in Bash are sequential lists that allow you to use variables or other string data types. They are very versatile and easy to work with. For example, we could create an array with 10 elements as follows: a1=1; a2=2; a3=3; a4=4; etc… If we wanted to loop through each element of the array, starting at 1 and ending at 10, it would look like this: for i in $(seq 1 10); do echo $i; done which outputs the following: 1 2 3 4 5 6 7 8 9 10
Accessing Values Within an Array
To retrieve the data stored in an associative array, a key must be supplied. This key can be any string of characters that uniquely identifies the desired value. A value is then found by running a loop through the values contained within the specified array. The variable used to iterate over the values (in this case i) is incremented with each iteration. The final step in accessing data from an associative array is performing an operation on it. In this example, when all four loops have completed, each element in fruits will have been incremented by one.
Destroying an Array
To destroy an array, the index can be reassigned or the unset function can be called. If you reassign the index, your new values will overwrite whatever was there before:
my_array=(my first string my second string my third string) $ my_array